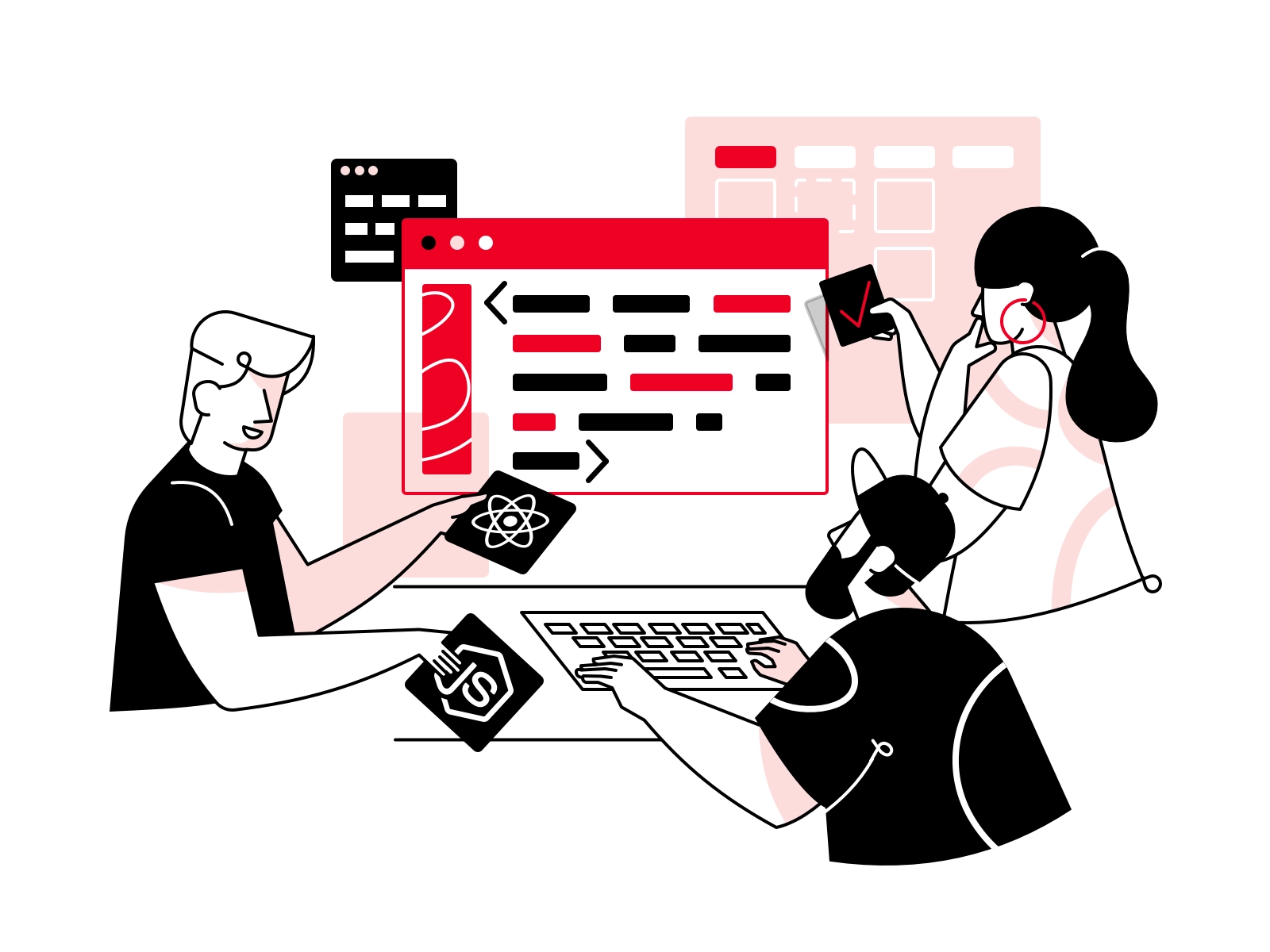
The What and Why of Node.js
Node.js is an open-source development platform that executes JavaScript code on the server-side. It is an asynchronous event-driven JavaScript runtime. It is designed to build scalable network applications. In summary, Node.js executes JavaScript programming language on the server side.
Node comes in handy in developing web-based applications that need a constant connection from the browser to the server. This is ideal for applications that need to be frequently updated, examples include: chat services, news feeds, and push notifications. In an ever-increasingly connected world, Node.js is a highly regarded choice.
How is Node.js Framework Created Differently?
As we stated above, Node has a more significant impact with fewer servers needed to get the job done. In traditional programming frameworks, the lag time within the app between the web server and the database can be lengthy. Node.js uses web server resources only on an as-needed basis, this means that it frees up a large number of resources for each user. Historically, more resources would be pre-allocated, creating a need for more server power. To put it succinctly, Node.js saves time and resources.
App Scalability and Why it Matters
Application scalability generally references an app’s capability to function well with growth. In more technical terms, scalability relates to the app’s backend, database and servers that host it. How can the databases and servers maintain performance with growing demand?
There are many approaches to maximizing scalability and maintaining performance levels both from an app development perspective and from an infrastructure standpoint. Ensuring app scalability is primarily the responsibility of the developers. Let’s take a look at performance factors for Node.js.
Node.js Performance
Performance can be boiled down to how quickly and accurately a web application responds to requests. It is one of the most important factors that a web-based product developer should consider as performance can make or break a product’s success. A fast, well-tuned application will make everyone involved happy as users and profits soar. And conversely, an app that is slow to load or deliver results will frustrate all involved parties.
However, speed and accuracy can be dependent on the number of users accessing the system at any given time. What works brilliantly for a thousand users, might not work so well for a million users. Scalability is important.
While Node.js is known for its performance and speed, there are tips for enhancing both as a Node.js built app scales.
Improving Node.js Performance at Scale
Node.js performance tuning helps ensure that an app runs at top levels at all times whether 10 users are engaged or 10 million. Read below for 8 tips to improve Node.js performance at scale.
8 Tips to Increase Node.js Application Performance
1. Test and Assess
Before diving in to make improvements on the performance of a product, it’s best to start by looking at how the system is functioning currently. Determining the inefficiencies as well as the product’s successes enables the right strategy adoption from the get-go. To properly assess the performance level, tests are generally required. Such tests might include but aren’t strictly limited to:
- Load Testing: Monitors the system’s response to increased load and how it adopts or handles changes in work.
- Stress Testing: Monitors how a system performs when pushed beyond normal limits. The goal of a stress test is to bring the system into failure and determine how much it takes before it fails and the duration necessary to recover from a failure.
- Scalability Testing: This test discovers the point when the app stops scaling and why.
- Endurance Testing: Monitors and evaluates the behavior of a software application under heavy load for a sustained duration. It is used to identify problems such as memory leaks.
2. Caching to Reduce Latency
Caching is a frequently used technique to improve app performance. It can be done both on the client and server side.
A cache is a high-speed storage layer that acts as a temporary warehouse for frequently accessed data. This way the data isn’t retrieved from a slower primary source but stays readily available in the cache. Storing frequently retrieved data in a cache prevents server resources from being redundantly occupied with repeating data retrievals. It is most efficient when being used for unchanging data.
Caching increases the speed of data retrieval, one of two ways, 1. by spending less time computing data or 2. by doing I/O (input-output). I/O involves fetching data over a network or from a database.
Enabling Asynchronous functions when using I/O will improve the apps functioning. The CPU will be able to handle multiple requests simultaneously without slowing I/O. If a request is making an I/O operation without asynchronous functioning it may block further processing until it completes the task at hand. This problem is eliminated with asynchronous functions.
Caching can also be used for API requests that are routed to an external server. If the API request responses can be reused for additional future requests, it makes sense to store them in a cache layer. This avoids future network requests and costs thereby associated with the API.
To implement caching in Node.js, a solution called node-cache is used. This puts actively and frequently used data into a memory where it can be accessed quickly.
In process caching, however, is tied to application processes. This means that it works poorly on distributed workflows. To remedy this, when a workflow is distributed, a caching solution such as Redis or Memcached can be used. Such solutions optimize the APIs response time by storing and retrieving the data from the main memory of the server. APIs with and without Redis show a huge difference in response time to queries: 899.37ms These programs run independently and function better when scaling an application onto multiple servers.
3. Minification
Another easy way to make sure an app runs efficiently and quickly is to focus on the front end. It’s imperative that anything sent to the browser is as small as possible; this is called minification. Size matters and this is particularly true for images, JavaScript, and CSS files.
Module bundlers are tools for processing groups of modules into smaller files. Module bundlers such as Webpack, Parcel, and Rollup, and task runners Gulp, and Grunt, are a few options. The results of the module bundlers or the minification process can be sent to production.
Complex transforms such as shortening multi-character variable names, shorter syntax and combining JavaScript files reduces the number of network requests. This also applies to CSS minification. The browser can parse more quickly because extra whitespace and comments are eliminated.
CSS preprocessors such as PostCSS, Sass, and LESS provide variables, functions, and mix-ins to reduce the maintenance necessary for CSS code. They also make refactoring easier. Last but not least, CSS processors compile all files into a single .css file. This sharply reduces the number of times the browser has to request a file from the server.
Images can be a beast that slows down apps; so the smaller or lighter the better. To meet these demands, images are often compressed or depending on the user’s device, an image may be selected over another. Gatsby is powered by Node.js and has a plugin library.
All bundlers mentioned run on Node.js.
4. Avoid Static Assets
Similar to the idea of minification of Java Script, CSS or images, avoid storing static assets that will hog space for optimal Node.js performance. When creating APIs in Node, avoid sending a full HTML page in a response. Node servers work optimally when exclusively data (JSON data) is sent by the API.
Node.js wasn't designed with this use case in mind. The job of storing static files is better handled by a web server such as Nginx or Apache, which performs optimizations better than Node.js. A CDN proxy can also be used to cache static content, freeing up Node.js servers to handle more dynamic requests.
5. Timeouts
A timeout is the predetermined maximum wait time ascribed to requests. It is the real-time of how long a client will wait before receiving a response from an external server. If a response doesn’t come within the allotted wait time, the connection aborts, and the application does not wait in limbo indefinitely.
Unfortunately, many popular libraries for making HTTP requests in Node.js (such as axios) do not set a maximum timeout. This means that a remote API could keep an application waiting for a response to a request indefinitely. Smart developers will set a request timeout to prevent this from slowing down an app. If even one piece in the chain is latent or unresponsive, and there’s no timeout set, end-users will receive a slow user experience.
6. Load Balancing
Load balancing is a solution used to distribute incoming traffic across multiple servers. Load balancers improve application availability and responsiveness and prevent server overload. Load balancers function as a go between for client devices and backend servers. They receive requests and then distribute them among available servers for fulfillment.
In a very neat function, Node.js can duplicate an application instance to handle more requests. This can be done on a single multicore server or through multiple servers.
Approach 1:
On a multicore server, Node.js can be scaled with the introduced cluster module. The introduced cluster module creates new processes that all run at the same time and connect to a single master process, allowing the processes to all share the same server port. This means that the cluster module behaves like one large multithreaded Node.js server. A round-robin strategy may be used to distribute incoming requests across the new processes (called workers) over multiple CPU cores.
Approach 2:
A second approach to load balancing is to use the PM2 process manager to keep applications running indefinitely. PM2 has a built-in load balancer and allows the application to run as multiple processes without code modifications. This helps avoid downtime that occurs when the app reloads due to a code change or error. Downtime is almost 0 with PM2. PM2 has a cluster feature that enables multiple processes to run across all cores without needing any code changes.
Approach 3:
Reverse proxying is one way to switch to load balancing across a multi-server environment. NGINX— a high‑performance, highly scalable, highly available web server, reverse proxy server, and web accelerator (combining the features of an HTTP load balancer, content cache, and more— supports load balancing across multiple Node.js servers and various load balancing methods. A reverse proxy feature protects the Node.js server from direct exposure to internet traffic.
7. Working with HTTP/2
Another simple way to make a Node.js application run quicker is to use HTTP/2, which improves performance and can solve issues that occur when using HTTP/1.x. The time necessary to create an HTTP connection is often longer and more costly to the system, than the time needed to transmit the data itself.
Two of the eye-catching features of HTTP/2 include:
- Header Compression – Removes unnecessary headers and puts all HTTP headers in a compressed format.
- Multiplexing- Enables multiple requests to retrieve resources and response messages in a single TCP connection simultaneously. Multiplexing minimizes the number of requests made to a server, which frees up resources.
To leverage HTTP/2, Transport Layer Security (TLS) and Secure Socket Layer (SSL) protocols need to be implemented. Node.js’ core implementation makes it very easy to set up an HTTP/2 server.
8. CDN Content Delivery Network
The time to the first byte TTFB is a tool used to measure the responsiveness of a web server or other network resource. It measures the duration from the user’s request initiation to the moment the first byte of the page is received by the client's browser. The TTFB can be reduced by using a CDN (Content Delivery Network— a geographically distributed group of servers that work together for rapid delivery of internet content) and caching content in local data centers. These steps help reduce latency. Cloudflare is a CDN solution.
Node.js System Service Final Thoughts
These tips are meant to make building an app in Node.js more user-friendly for the end user and create a great user experience that keeps them coming back to the app. With all this information in mind, however, one key factor to remember is that reliability is not worth trading out for performance.
A key element: the security of routes must be optimized and tested. A speedy but insecure API response is for all intents and purposes futile. It’s important to stay vigilant and keep security checks at a maximum while building in Node.js. A balance between Node.js performance tuning, security, development, and continued maintenance costs is ideal.
Finding the Right App Development Partner
Finding the right development partner can be tricky. There are many factors to consider that go into a great partnership, including your budget and time-frame. You also want to ensure your development partner has experience in delivering projects similar to yours, the ability to provide tailored solutions that fit your unique business needs, and that there is a culture fit between companies and employees. We have an entire article dedicated to helping you find the right cultural fit for your development needs.
At Pixel506, our years in the industry and development experience have earned us accolades and recognition. We work with clients to make sure every project is custom-tailored to their needs and desires. Our nearshore status makes our services competitively priced. Our talented developers are experienced in Node.js and we are an end-to-end agency, capable of working with our clients past the development phase and into ongoing support and maintenance, product launches, and lifecycles.
Key Takeaways
- Node.js is an open-source development platform that executes JavaScript code on the server-side.
- Steps to increase Node.js application performance include testing and assessing, caching to reduce latency, minification, avoiding static assets, timeouts, load balancing, and working with HTTP/2.
- A balance between Node.js performance tuning, development, and continued maintenance costs is ideal.
- Factors to pay attention to when choosing an app development partner include your budget and time-frame, and the potential partner’s talent, experience and company culture.
About Pixel506
Pixel506 is an award-winning nearshore agency that helps companies succeed in the digital world. We develop websites, design mobile applications, provide social media support, create content, conduct keyword research, set up SEO and SEM campaigns, and design customized brand styles from the ground up—and our engineers and developers are versed in Node.js to help make real-time applications function with ease.
Since 2009, we have continuously expanded our knowledge, skills, and experience. Today, as an end-to-end marketing agency, Pixel506 offers a wide variety of services across the creative, digital and technological landscape. Our people-focused, data-driven, and results-oriented philosophy ensures that everything we produce is wholesome, measurable, and efficient.
We invest heavily in developing and maintaining strong client relationships. By working closely with our clients, we ensure that we offer customized solutions and deliver high-quality, insightful, and actionable results. Most of our team members are located in Latin America, but we are a Brooklyn-born company with a strong client base in North America.Are you considering software development nearshoring? Looking for a reliable partner agency? Interested in learning more about our services? Leave us a message and we’ll be sure to get back to you as soon as possible.